An absolute path specifies the entire path from the root directory of the file system to the file. It is independent of the current script's location.
In contrast, a relative path specifies the path to the file based on the directory of the current script. It varies depending on the current location of the script.
Absolute Path
This is a path that begins from the root (/, ROOT) directory, the highest directory on the server.
For example, '/var/log/mail/' or 'C:\Users\Username\Documents' in Windows.
Relative Path
This is a path that is specified relative to the directory you are currently in. The current directory is denoted by '.', and the parent directory is denoted by '..'.
If you have the directories '/var/log/mail/' and '/var/home/', the relative path from the current '/mail/' location to '/home/' is '../../home/'. Also, the relative path from '/log/' to '/mail/' is './mail/'.
PHP Example Code
You can use built-in PHP functions to check the absolute and relative paths of the file where the current code is written in various ways.
// Absolute path of the current script
$currentPath = realpath(__DIR__);
// Absolute path of the parent directory
$parentPath = realpath($currentPath . '/..');
// Absolute path of another directory
$otherPath = realpath($currentPath . '/path/to/other/directory');
// Relative path between the current script and another file
$relativePath = 'path/to/another/file.txt';
$relativeToCurrent = realpath($currentPath . '/' . $relativePath);
// Relative path between the parent directory and another file
$relativeToParent = realpath($parentPath . '/' . $relativePath);
// Output
echo "Current Path: " . $currentPath . "\n";
echo "Parent Path: " . $parentPath . "\n";
echo "Other Path: " . $otherPath . "\n";
echo "Relative to Current: " . $relativeToCurrent . "\n";
echo "Relative to Parent: " . $relativeToParent . "\n";
Absolute and Relative Paths in 'include' and 'require'
In PHP, include and require are used to insert the contents of one PHP file into another before the server executes it.
// Absolute path
include '/var/www/html/includes/header.php';
require '/var/www/html/includes/footer.php';
// Relative path
include 'includes/header.php'; // Relative to the current directory
require '../common/footer.php'; // Relative to the parent directory of the current directory
In the example, '/var/www/html/includes/header.php' and '/var/www/html/includes/footer.php' are absolute paths. They specify the full path from the root directory (/) to the file.
'includes/header.php' is a relative path. It looks for the 'header.php' file in the 'includes' directory located in the same directory as the current script. '../common/footer.php' navigates one step up (..) in the directory structure and then finds the 'footer.php' file in the 'common' directory.
Comparison of Absolute and Relative Paths
Aspect | Absolute Path | Relative Path |
---|---|---|
Portability | Low portability due to being specific to the server's directory structure. | High portability as it is relative to the script's location, making it easier to move the codebase to other servers or environments. |
Maintainability | Difficult to maintain as you need to update paths each time the directory structure changes. | Easy to maintain under a consistent directory structure. If the script's location changes, relative paths adjust automatically. |
Usage Context | Useful when the exact location of the file is fixed and certain, such as when including configuration files or external libraries. | Useful when including files within a project with a consistent directory structure. |
Absolute Path Using '__DIR__'
include __DIR__ . '/includes/header.php';
require __DIR__ . '/includes/footer.php';
__DIR__ is a magic constant in PHP that provides the directory of the current file. This approach combines the portability of relative paths with the stability of absolute paths within a project.
Choosing between absolute and relative paths depends on the specific requirements of the project. Relative paths are generally preferred for internal inclusion within the same project due to their portability and ease of maintenance. Absolute paths can be more reliable for important configuration files or external libraries.
PHP Reference Pages
PHP: Hypertext Preprocessor
https://www.php.net
PHP GD Library, JPEG PNG Lossy Compression Ratio
https://www.ppcle.com/post/php-gd-library/
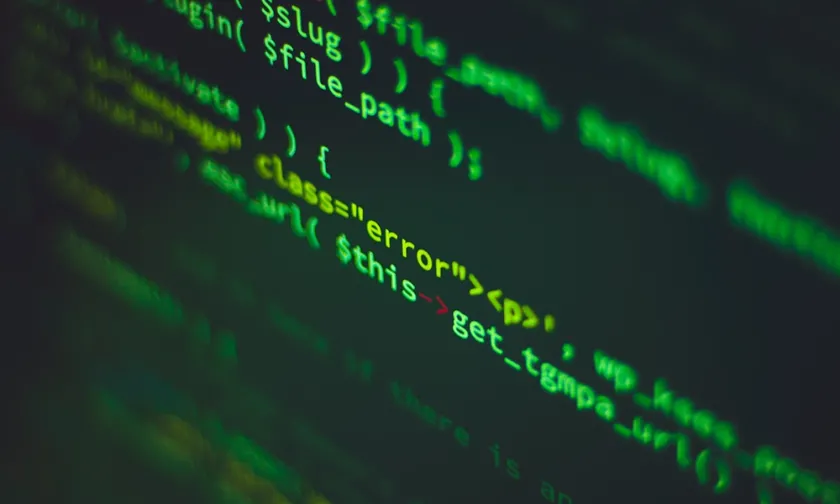